5 Reasons Why You Should Use Data Class in Python
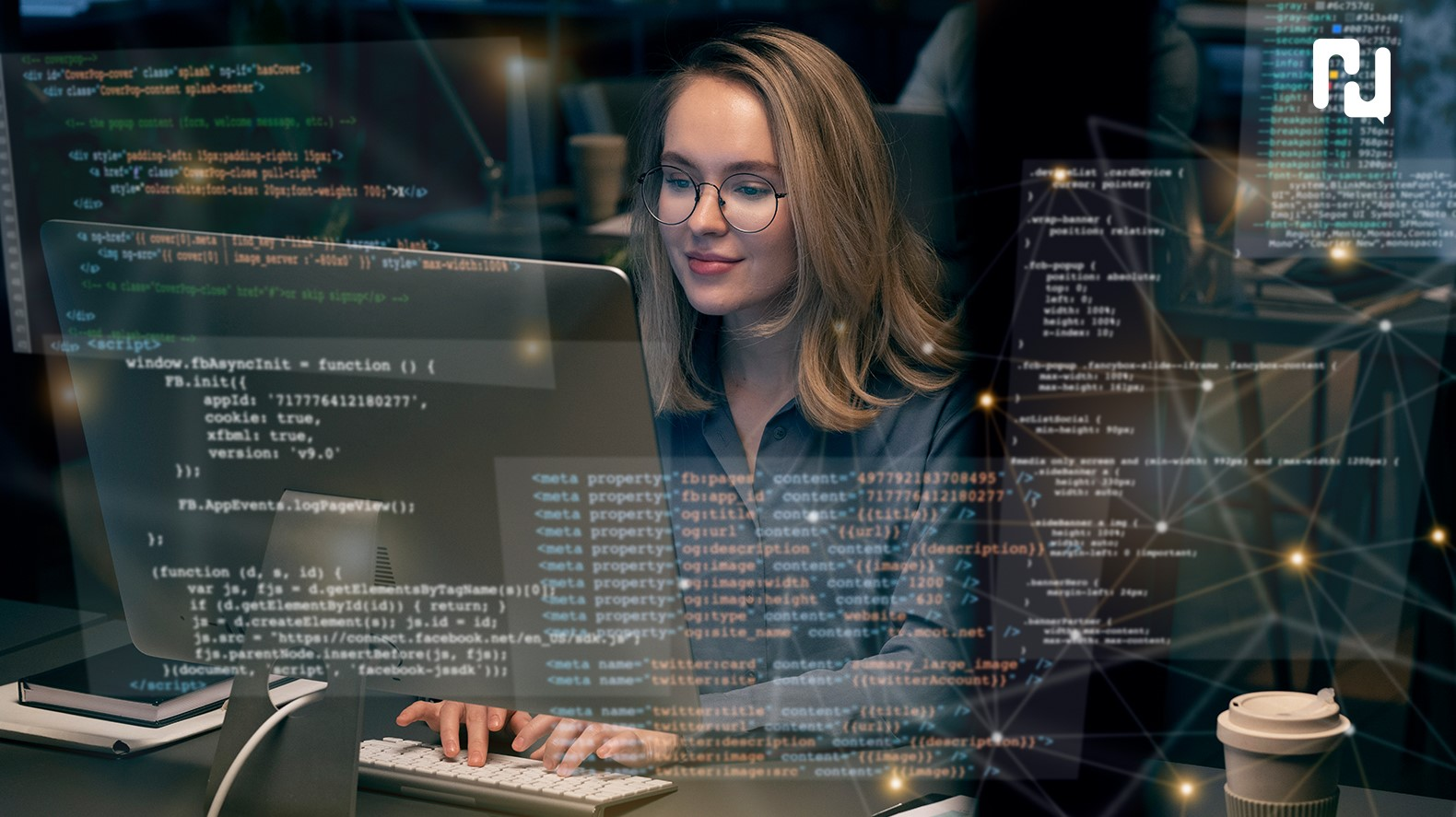
Data handling is a fundamental aspect of programming in Python. Data classes are powerful and convenient. They allow developers to create classes primarily for storing data without writing boilerplate code. Python class as a data container simplifies the process of defining classes to be used primarily as data containers. In this article, we will explore five compelling reasons why you should embrace data classes in Python.
What is a Data Class in Python
In Python, a data class is a class that is primarily used to store data, and it is designed to be simple and straightforward. Data classes are introduced in Python 3.7 and later versions through the data class decorator in the data classes module.
The purpose of a Python data class is to reduce boilerplate code that is typically associated with defining classes whose main purpose is to store data attributes. With data classes, you can define the class and its attributes in a more concise and readable manner.
How to Use Data Class in Python
To create a data class, you simply need to decorate the class with the @dataclass decorator and specify the attributes you want to store as class variables. Python will automatically generate several special methods like __init__, __repr__, and __eq__ for you based on the attributes defined.
Why You Should Use Data Class in Python
-
Simplified Class Definitions
Traditional class definitions in Python can quickly become verbose and repetitive when dealing with data storage. With data classes, you can significantly reduce the amount of code needed to define a class for holding data. By simply adding the @dataclass decorator from the data classes module, Python automatically generates special methods like __init__() and __repr__(). This is how python dataclass saves development time but also enhances code readability and maintainability.
-
Automatic Initialization
Data classes automatically create class instances. You don't need to explicitly define an __init__() method to set attributes during object creation. This automatic initialization allows you to create instances of the data class with concise and clean syntax, making your code more elegant and less prone to errors.
-
Inbuilt Comparison Methods
Python's data classes come with built-in methods for comparison, such as __eq__(), __ne__(), and __lt__(). These Python data class methods enable instances of the data class to be compared based on their attributes. This can be extremely useful when you want to compare objects for equality or sorting purposes, enhancing the functionality of your code.
-
Immutability and Immutable Defaults
Data classes offer options for creating immutable objects. Using the frozen=True parameter with the @dataclass decorator, you can make instances of the class immutable, preventing accidental modifications. Additionally, you can define default values for attributes, which further simplifies the code by eliminating the need for explicit default values in the __init__() method.
-
Simplified Data Modification
When working with data classes, you can easily modify the attributes of an instance using the replace() method. This method creates a new instance with the desired changes, leaving the original instance unchanged. This approach makes your code more predictable and safer, as it avoids unintentional side effects while modifying data.
Limitations of Using Data Class in Python
Using data classes in Python can be very convenient for creating simple classes with a lot of boilerplate code automatically generated. However, they do have some limitations:
Immutability
By default, data classes are mutable, which means you can modify their attributes after instantiation. This might not always be desirable, especially in cases where you want to ensure that the data remains constant once created. To enforce immutability, you need to add extra code or use additional libraries.
Limited customization
While data classes provide automatic generation of special methods like __init__, __repr__, __eq__, and more, customizing their behavior can be challenging. If you need more fine-grained control over these methods, you might be better off writing the class manually.
Inheritance
Data classes don't play well with inheritance. If you want to create a subclass of a data class, it can become complicated, and certain behaviors might not work as expected. This limitation can sometimes be overcome using abstract base classes or more complex workarounds.
Namespace pollution
Data classes automatically generate __init__, __repr__, and other methods, which can clutter the class namespace. In situations where you have many attributes, this can make it harder to work with the class directly.
Lack of post-init validation
Data classes provide no built-in mechanism for performing validation on attribute values during instantiation. If you need to ensure that specific conditions are met before setting an attribute, you'll need to implement that logic separately.
Compatibility with older Python versions
While data classes are a standard feature in Python 3.7 and above, they are not available in older versions. If you need to support legacy Python codebases, using data classes might not be an option.
JSON serialization
Data classes do not provide built-in JSON serialization out-of-the-box. If you need to serialize your data objects to JSON, you will need to implement a custom solution or use external libraries.
Despite these limitations, data classes are a powerful tool for many use cases, especially when working with simple, data-focused classes, and they can significantly reduce boilerplate code. However, for more complex scenarios or when specific customization is needed, you might need to consider traditional classes.
Coping With the Limitations
Coping with the limitations of data classes in Python can be achieved by employing different strategies and using additional tools. Here are some approaches to address each limitation:
Immutability: To enforce immutability, you can use the frozen attribute in the data class decorator. By adding frozen=True, you prevent modifications to instances after instantiation.
Limited customization: For more customization, consider creating traditional classes instead of data classes. By writing your methods explicitly, you have full control over their behavior and can tailor them to your needs.
Inheritance: When dealing with inheritance, using traditional classes or abstract base classes (ABCs) might be a better choice, as they provide more flexibility and predictability in the inheritance hierarchy.
Namespace pollution: If you are concerned about namespace pollution, use the data class decorator with the frozen attribute, as mentioned in the first point, to make the class immutable. Additionally, you can consider organizing your code in modules or packages to manage namespaces better.
Validation: To handle validation during instantiation, you can create a custom __post_init__ method. This method will run after regular __init__ and allows you to add validation logic.
Compatibility: If you need to support older Python versions, you can use the data classes module from the standard library, which provides a backport of data classes for Python 3.6 and earlier.
JSON serialization: To enable JSON serialization for data classes, you can use external libraries like dataclasses-json, attrs, or marshmallow.
By being aware of these limitations and employing the appropriate solutions, you can make the most of data classes while overcoming their drawbacks. It's essential to choose the right tool for the job and adapt your approach based on the specific requirements of your project.
Conclusion
In conclusion, data classes in Python offer a plethora of advantages that make them indispensable for handling data in a concise and efficient manner. The simplicity and elegance they bring to class definitions, along with automatic initialization, inbuilt comparison methods, immutability options, and simplified data modification, significantly enhance the overall development experience. By utilizing data classes, you can write cleaner, more maintainable, and error-resistant code.
If you're looking to take your Python programming skills to the next level and explore the exciting world of block chain, look no further! Our blockchain services provide comprehensive guidance, training, and hands-on experience to help you build cutting-edge applications on decentralized networks. Unlock the potential of blockchain technology and join our community today!